Introduction:
After the announcement of Google on Google I/O 2017, we
have seen the series of tutorials in Kotlin. If you are new to Kotlin you can
learn Kotlin from our previous articles. We had been crossed Basics of Kotlin and Hello World
function, How to use ListView, RecyclerView in Kotlin and How to implement
SQLite storage in Kotlin with CRUD operations.
In this article, we will learn how to make server call
(i.e. HTTP Connection) from Kotlin powered application to web services.
Coding
Part:
I have divided the coding part into 3
steps as shown in the following.
- Creating new project.
- Setting up the project with Fuel HTTP.
- Implementing the Fuel HTTP Service Call.
Let’s start coding for Fuel HTTP.
Full Code of MainActivity:
Step
1: Creating new project:
- Open Android Studio and Select Create new project.
- Name the project as your wish and tick the Kotlin checkbox support.
- Then Select your Activity type (For Example: Navigation Drawer Activity, Empty Activity, etc.).
- Then Click “finish” button to create new project in Android Studio
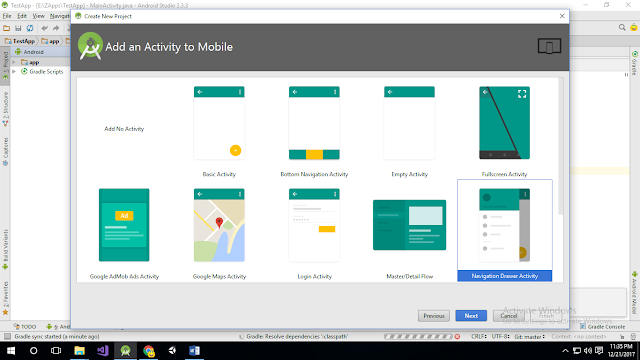
Step 2: Setting up the project:
Now, we
will setting up the project for Fuel HTTP.
1.
Open your
app level build.gradle file
and add the following line.
implementation 'com.github.kittinunf.fuel:fuel-android:1.6.0'
2.
If you are using Android Studio
Version below 3.0, the add the following (Optional
Step)
compile 'com.github.kittinunf.fuel:fuel-android:1.6.0'
3.
Then Click “Sync Now” to
download the library and add to the project.
After this, you are ready to
implement HTTP Service Call from your Application.
Step 3: Fuel HTTP implementation:
Step 3: Fuel HTTP implementation:
Now, we
will setting up the project for Fuel HTTP.
1.
Open your AndroidManifest.xml
and Add permission to access internet.
<uses-permission android:name="android.permission.INTERNET"/>
2. Open your activity_main.xml
file and create the User Interface as you wanted or Paste the following code.
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.androidmads.kotlinfuelhttpsample.MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:text="GET Request's Response"
android:textColor="#000000" />
<TextView
android:id="@+id/tvGetResponse"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:background="#e1e1e1"
android:padding="10dp"
android:text="" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:background="@color/colorPrimary"
android:onClick="httpGetJson"
android:text="GET RESPONSE"
android:textColor="#ffffff" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:text="POST Request's Response"
android:textColor="#000000" />
<TextView
android:id="@+id/tvPostResponse"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:background="#e1e1e1"
android:padding="10dp"
android:text="" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:background="@color/colorPrimary"
android:onClick="httpPostJson"
android:text="POST RESPONSE"
android:textColor="#ffffff" />
</LinearLayout>
</ScrollView>
Layout Preview
1. Then Open your Activity file and here I am opening MainActivity.java file.
2.
Initialize the fuel library by
the following code
FuelManager.instance.basePath = "http://demosmushtaq.16mb.com";
Here, you must specify the base
address of your service. It will start or initialize the library.
GET REQUEST
We will use “Fuel.get()”
to make server call with Get method. The following code shows how to implement
this.
Fuel.get("<Service Link without base path>")
.responseJson { request, response, result ->
Log.v(“response”, result.get().content)
}
POST REQUEST
We will use “Fuel.post()”
to make server call with Post method. The following code shows how to implement
this.
Fuel.post("<Service Link without base path>", listOf("<key>" to "<value>"))
.responseJson { request, response, result ->
Log.v(“response”, result.get().content)
}
You can get the response as string or json by specify the response type “responseString” or “responseJson”
respectively.You can pass the data to service
using “listOf” method. It is an
optional value. You can also access the without the parameters to be passed
You can get response from the result
using “result.get().content” as
mentioned in the code above.
Full Code of MainActivity:
You can find the full code implementation of
MainActivty.kt in the following.
class MainActivity : AppCompatActivity() {
var tvGetResponse: TextView? = null
var tvPostResponse: TextView? = null
var progress: ProgressDialog? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
initViewsAndWidgets()
FuelManager.instance.basePath = "http://demosmushtaq.16mb.com";
}
private fun initViewsAndWidgets() {
tvGetResponse = findViewById(R.id.tvGetResponse)
tvPostResponse = findViewById(R.id.tvPostResponse)
progress = ProgressDialog(this)
progress!!.setTitle("Kotlin Fuel Http Sample")
progress!!.setMessage("Loading...")
}
fun httpGetJson(view: View) {
try {
progress!!.show()
Fuel.get("api/get_sample.php").responseJson { request, response, result ->
tvGetResponse!!.text = result.get().content
}
} catch (e: Exception) {
tvGetResponse!!.text = e.message
} finally {
progress!!.dismiss()
}
}
fun httpPostJson(view: View) {
try {
progress!!.show()
Fuel.post("api/post_sample.php", listOf("version_index" to "1")).responseJson { request, response, result ->
tvPostResponse!!.text = result.get().content
}
} catch (e: Exception) {
tvPostResponse!!.text = e.message
} finally {
progress!!.dismiss()
}
}
}
Download Code:
You can
download sample code from the following GitHub link.